京都の外壁塗装で選ぶべき評判・口コミの良い業者ランキング【2021年】
京都の外壁塗装業者を徹底調査!
京都で評判の良いおすすめ外壁塗装業者ランキング!
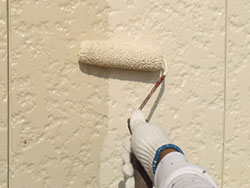
こんにちは、「京都のおすすめ外壁塗装業者を実績・評判から厳選して10社ご紹介」の管理人です。
この度は当サイトをご覧いただきき、本当にありがとうございます。
このサイトでは、京都で生まれ育った管理人である私が、地元である京都の優良外壁塗装業者をご紹介しています。
京都にお住まいの方で、外壁塗装を検討されている方にとって少しでもお役に立てれば幸いです。
まず、なぜ私が「京都の優良外壁塗装業者」を紹介しようと思ったのか? 手短にご説明させていただきます。
実は最近、たくさんの思い出が詰まった我が家の外壁塗装工事を行いました。
築年数は約20年程。
築20年を超えたくらいのタイミングで塗り替えをしないと家の寿命が短くなってしまうと聞いたこと。
そして何より、この20年一切外壁には手をかけていなかったので、汚れが目立ち美観がすごく悪くなっていたこと。
これが初めて外壁塗装の塗り替えを検討するきっかけとなりました。
私は性格的に凝り性で、何でも自分で調べないと気が済まない性格です。
自分が納得するまで徹底的に調査して、どこよりも良い塗装業者さんを見つけて塗り替えをしてもらおう!と意気込みました。
幸いにも、私は職業柄、そして長年京都に住み続けていることもあり、建築関係(大工さん・建築士さん・設計士さんなど)の知人が複数います。
実際に行った調査方法として、その建築関係の知人と直接会い、徹底的に塗装業界を含む建築業界の生の声を聞き込みました。
その結果、納得・安心して塗り替えをお願いできる塗装業者さんと出会うことができ、仕上がり・工事金額ともに満足のいく塗り替えをすることができました。
私と同じように、
・大切な家だからこそ、丁寧にしっかりと外壁塗装をしてほしい
・少しでも安くなれば嬉しいけど、手抜き工事はされたくない
・しっかり工事内容を説明してくれるような、信頼できる塗装業者を見つけたい
とお考えの方は、きっとこのサイトが京都での塗装業者探しの参考になると信じております。
ぜひ、京都にお住まいの方で、外壁塗装を検討されている方、優良塗装業者をお探しの方の参考にしてください。
京都で信頼できる外壁塗装業者を選ぶポイント
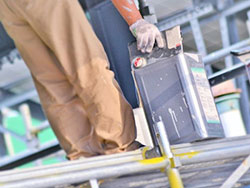
今回、私が「優良外壁塗装業者の定義」として挙げたポイントは以下の3点です。
① 良心的な外壁塗装の金額設定
② 塗り替えの施工技術のレベルが高い
③ 対応品質・サポートがしっかりしている
恐らく皆さんにとっても、上記3点が揃っていれば優良塗装業者と言えるでしょう。
しかし、京都にも外壁塗装業者は数多くありますが、上記全てを満たす塗装業者というのは実は非常に少ないのです。
「値段が安くても、塗装工事自体の品質が悪い」
「仕上がりはすごく良いけど、工事金額が相場より明らかに高額」
「会社の規模は大きいが、施工は全て下請けに丸投げで対応が雑or下請け任せ」
など、どれか1つの項目は満たしても、他のポイントが良くないという塗装業者が圧倒的に多いのが現状です。
それでは、「優良外壁塗装業者の定義」をもう少し掘り下げてご説明します。
良心的な外壁塗装工事の金額設定
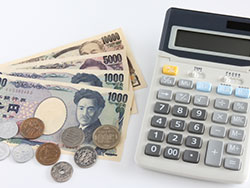
良心的な金額設定は、やはり皆さん重視したいポイントではないでしょうか?
私自身もここは絶対に外せないポイントでした。
一般的な戸建住宅の外壁塗装の価格相場は、おおよそ80万〜120万円とされています。
しかし、ただ安ければ良いという訳でない、施工品質が伴わないといけない、というのが難しいポイントです。
良心的な工事金額、その中で最大限高品質な塗り替えを行ってくれる塗装業者を見極めるポイント。
それは、
営業マンやCM・チラシなど、過剰な営業費・宣伝広告費を使っていないかどうか、です。
外壁塗装業界は、大手メーカーから地元密着型の小さな塗装業者まで、お客さんの奪い合い状態となっています。
そのため、多くの塗装業者が他社より少しでも知名度を上げるため、少しでも多くのお客さんに知ってもらうために、多額の営業費・宣伝広告費を使っています。
大手メーカーであればCM、小さな塗装業者でも新聞チラシ、そして多くの営業マンを雇い、訪問販売による営業活動を行なっているのです。
もちろん塗装業者も生活がかかっているので、営業・宣伝を行い、少しでも仕事を受注しようとするのは当然です。
しかし問題は、多額の営業費・宣伝広告費は皆さんがお支払いになる工事代金に含まれる、という点です。
過剰な営業費・宣伝広告費を使っている塗装業者は、総じて塗り替えの工事金額が高額になりがちです。
こうなると、「なぜ私たちが工事には直接関係の無い営業費・宣伝広告費を支払わないといけないのか」と思ってしまいますよね。
本当に優良な塗装業者なら、広告を出したり営業マンを雇ったりしなくても評判・口コミでお客さんは自然と集まるのです。
ただでさえ価格相場の幅が広く、工事金額の内訳がわかりにくい外壁塗装です。
気になった塗装業者が、営業マンを雇っていないか、過剰な広告を出していないか、確認してみましょう。
塗り替えの施工技術のレベルが高い
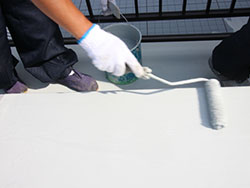
施工技術・施工品質に関しては、気にはなりますがわかりにくいポイントです。
私もそうでしたが、普段塗装工事に馴染みが無い一般の方からすれば、実際に施工している姿を見ても、技術の良し悪しを見極めるのは難しいのです。
ここで1つの見極めポイントとなるのが、「一級塗装技能士」という資格です。
この「一級塗装技能士」は、塗装の実技試験と学科試験の両方に合格した塗装職人のみが持っている資格になります。
もちろんこれは国家資格です。
資格を持っていない塗装職人さんでも、技術力が高い職人さんは沢山いらっしゃると思いますが、少なくともこの資格を持つ塗装職人さんは、一定水準以上の技術・知識を持つ塗装職人だと国から認められているのです。
気になった塗装業者に「一級塗装技能士」の取得者がいるのか、ぜひ確認しておきましょう。
塗装工事以外にも接客対応・サポートがしっかりしている
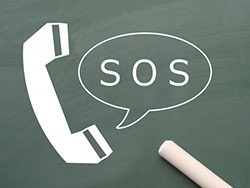
外壁塗装を検討されている方の中で、外壁塗装について精通してるという方は少ないと思います。
なぜなら、外壁塗装の塗り替え周期は10~20年に一度、知らなくても当然なのです。
むしろ私のように、「初めて外壁塗装を検討している」という人の方が多いかもしれません。
一般の方が馴染みが薄い外壁塗装工事だからこそ、対応品質・サポートがしっかりしている塗装業者の方が安心して施工を任せられるでしょう。
打ち合わせや現地調査、見積もりの段階で、「わからないことはないか?心配な点はないか?」など、気遣ってくれる塗装業者は本当に心強いです。
初めて塗り替え工事を行う方なら、尚更安心して工事を任せることができます。
こちらの些細な疑問・質問にも、丁寧にしっかり対応してくれる塗装業者を選ぶようにしましょう。
また、不思議と対応品質が良い塗装業者は、総じて塗り替えの施工品質も高いです。
やはり、真面目に誠実に塗装と向き合っている業者は、その真面目さ・誠実さが対応品質にも現れるということですね。
京都で評判の良い外壁塗装業者はどこ?
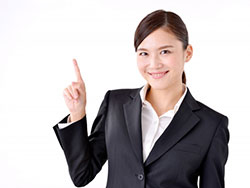
以上のことを踏まえた上で私は、複数の塗装業者から見積もりをいただき、じっくりと見積書を比較検討し、塗装業者を吟味しました。
そしてその結果、京都の中でも大変素晴らしい外壁塗装業者さんと出会うことができ、仕上がり・価格ともに満足のいく外壁塗装工事をしていただくことができました。
ここでは、私が実際に塗り替えを依頼した塗装業者さんをはじめ、
・見積もりを依頼したり問い合わせたりした際に「3つの優良外壁塗装業者の定義を満たしている」と感じた塗装業者さん
・建築業界に在籍している知人の中で評判の良い塗装業者さん
この2点を含めた、京都で安心して塗装工事を任せることができる優良外壁塗装業者さんをランキング形式でご紹介させていただきます。
ぜひ京都にお住まいの方は、優良外壁塗装業者探しの参考にしてください。
京都で評判・口コミの良い外壁塗装業者ランキング
ランキング1位

株式会社ウェルビーホーム
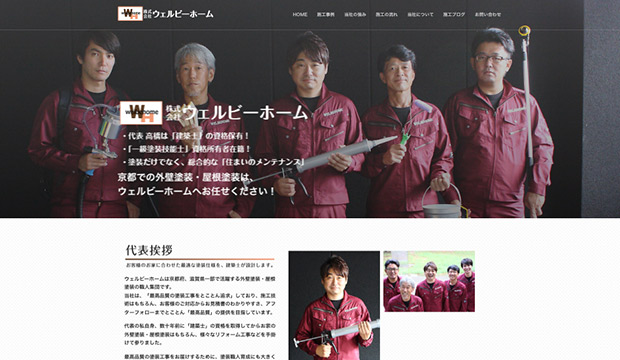
株式会社ウェルビーホームさんの特徴
今回、私が実際に外壁塗装の塗り替えを依頼し、最も皆さんにもおすすめしたい外壁塗装業者さんは、「株式会社ウェルビーホーム」さんです。
ウェルビーホームさんは、代表の高橋さんが直接打ち合わせ・現場調査から実際の施工、そして工事後のアフターフォローまで全て対応してくれます。
また、高橋さんは建築士の資格も持っており、外壁塗装だけでなく住まいのこと全般に精通しておられるので、様々な観点から外壁塗装に関するアドバイスをしてくれます。
高橋さん自身とても笑顔の素敵な物腰の優しい方で、外壁塗装以外の住まいに関する質問にも、とてもわかりやすく丁寧に答えてくださいました。
見積書も、他のどの業者さんよりも丁寧で詳細に作ってくださり、工事内容が非常に明確で安心できたのがウェルビーホームさんを選んだ大きなポイントです。
さらに、ウェルビーホームさんは「一級塗装技能士」の資格を所持する職人さんが在籍しています。
施工してくれた職人さん方もとても愛想良くマナーもしっかりしており、塗り替えの仕上がり・接客応対ともに大満足の結果でした。
工事金額も他業者さんと比べても安価でしたし、是非とも京都で外壁塗装を検討されている方におすすめしたい優良塗装業者さんです。
株式会社ウェルビーホームさんを利用した方の評判・口コミ
40代・女性・京都市中京区在住
外壁塗装が初めての私にも非常に丁寧にご説明くださいました。
打ち合わせの時点から高橋社長の人柄の良さは伝わりました。
見積書の内容も細かく教えてくださり、初めての外壁塗装も安心して行えました、ありがとうございました。
50代・男性・京都府城陽市在住
問い合わせから施工までやりとりがとてもスムーズでした。
特に問い合わせから現地確認までの対応の早さは一番でした。
カラーシミュレーションなど、色決めも早かったです。
仕上がりも綺麗で大満足です。
50代・女性・大阪府寝屋川市在住
前回の工事代金より大幅に安かったので大変驚きました。
こんなことなら前回の塗装工事もウェルビーホームさんにお願いしたかったです。
次回の塗装もお願いしたいので、その際はよろしくお願いします。
京都で評判・口コミの良い外壁塗装業者ランキング
ランキング2位

株式会社 佐藤塗装店
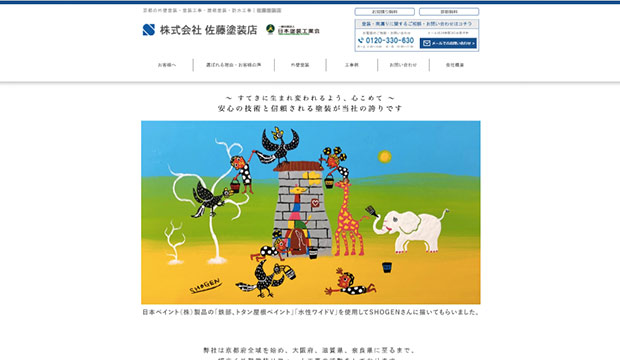
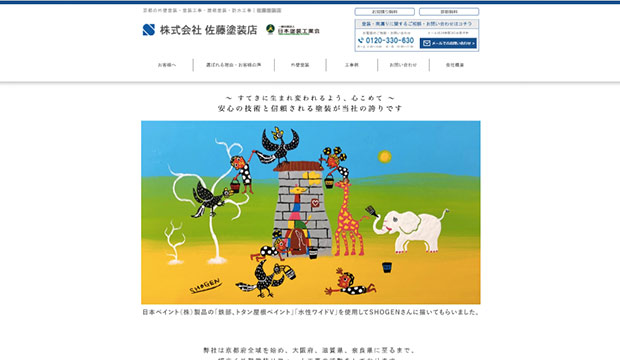
株式会社 佐藤塗装店さんの特徴
「株式会社 佐藤塗装店」さんは、京都市西京区を中心に京都府全域、大阪府、滋賀県、奈良県と幅広いエリアで活躍する塗装業者さんです。
代表の佐藤さんは、「一級塗装技能士」の資格をはじめ、「一級左官技能士」「足場組立作業主任者」など、数多くの資格を有しておられます。
塗り替えを依頼する塗装業者さんが塗装だけでなく他の建築工事に関して精通していると、幅広い視点で的確なアドバイスをもらえるので嬉しいポイントです。
佐藤塗装店さんは、日本ペイント株式会社の特約店であり、使用する塗料も日本ペイントが主になりますので、塗料の単価や性能など、工事内容が非常に明確です。
見積もりの内容も分かりやすく明瞭会計で、職人さんの対応も非常に丁寧です。
京都の数ある塗装業者の中でも、5指に入る優良塗装業者さんです。
株式会社 佐藤塗装店さんを利用した方の評判・口コミ
50代・男性・京都市西京区在住
日本ペイントの塗料と聞いて安心しましたし、塗料の知識が豊富な営業さんで、勉強になりました。
価格がわかりやすく、日本ペイントの塗料を使用しているとのことで、決めました。
仕上がりにも大変満足です。
50代・女性・兵庫県神戸市在住
住まいのイメージを変えたく、相談に乗っていただきました。
熱心に対応して頂き、次回もお願いしたいと思います。
ありがとうございました。
40代・女性・大阪府堺市在住
塗装後玄関周りの色が気に入らず、再度塗り替えて頂き有難うございました。
大満足です。ご近所様をご紹介させて頂きます。
京都で評判・口コミの良い外壁塗装業者ランキング
ランキング3位



株式会社 伊藤建装
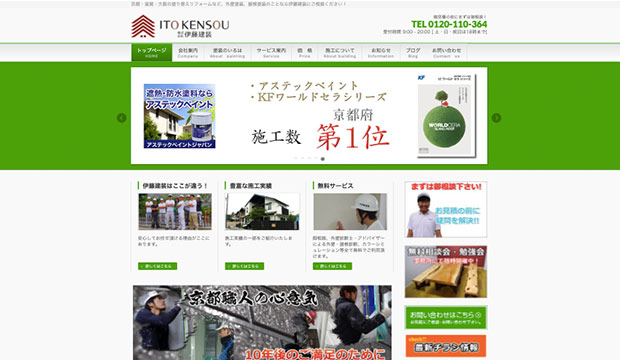
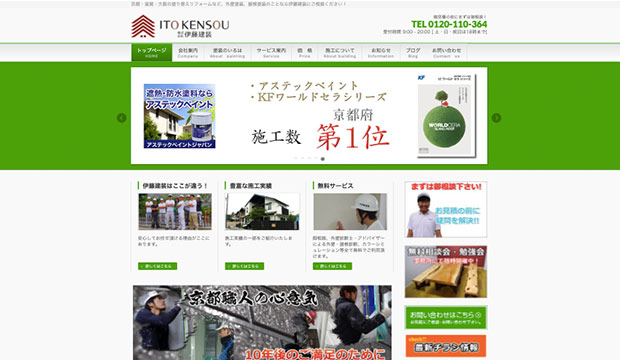
株式会社 伊藤建装さんの特徴
「株式会社 伊藤建装」さんは、京都市伏見区を中心に京都府・滋賀県・大阪府北部で活躍する塗装業者さんです。
「一級塗装技能士」の資格保有者だけでなく、外壁診断士や外壁アドバイザーなどの資格保有者が複数在籍しておられ、まさに外壁のプロフェッショナルです。
非常に多くの塗料・プランをご用意されており、外壁診断士さんがお住まい一軒一軒に合ったプランを提案してくれます。
非常に多くの職人さんが在籍され、宣伝・広告にも力を入れておられるので、工事金額自体は決して安価ではありません。
しかし、外壁をしっかり診断してほしい・質の良い塗装工事をしてほしいといった、金額より施工品質重視の方にはおすすめの塗装業者さんです。
株式会社 伊藤建装さんを利用した方の評判・口コミ
40代・女性・京都市左京区在住
職人さん達はゴミの片付けもきっちりされていて良かったと思います。
女性の職人さんがいらっしゃったので驚きました。
足場を組む時なども男性と変わらない力強さで仕事をこなされていたので感心してました。
40代・男性・京都市西京区在住
細かいところまで綺麗にして頂き、ありがとうございました。
工事中、台風が来た時には困りましたが、結果的に綺麗に仕上げてくださり満足です。
50代・男性・滋賀県大津市在住
見積もりから施工まで、こまかいこだわりを重視してくださりありがとうございました。
家が生まれ変わったように綺麗になり感謝しています。
丁寧な職人さん達にも感謝です。